In this blog post we will Provide Coding Ninjas Basics of Java with Data Structures and Algorithms Solution. This will have solutions to all the problems that are included in Coding Ninjas Java Course. Given a number N, print the following pattern Coding Ninjas question with solution.
Coding Ninja question
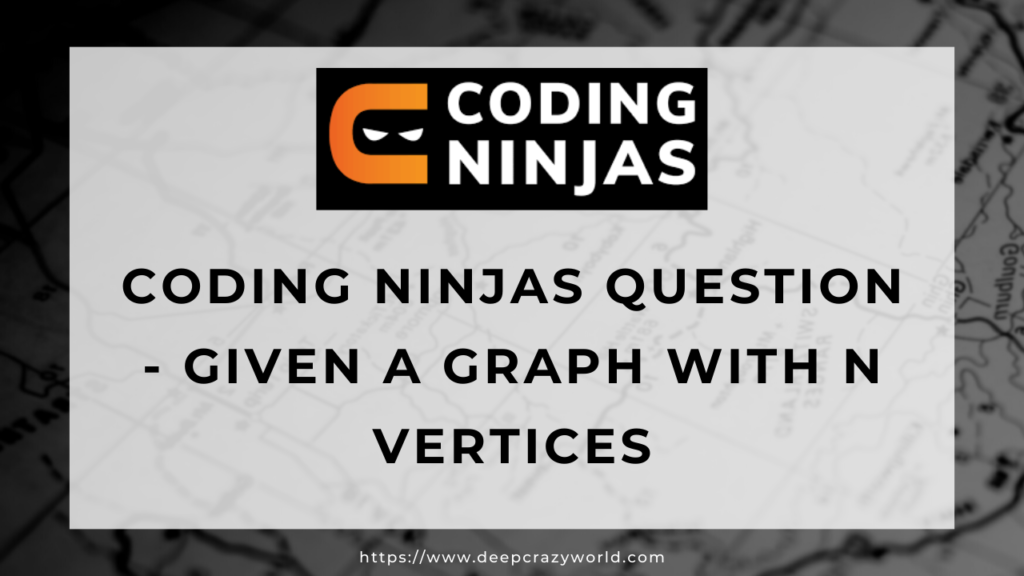
Given a graph with N vertices (numbered from 0 to N-1) and M undirected edges, then count the distinct 3-cycles in the graph. A 3-cycle PQR is a cycle in which (P,Q), (Q,R) and (R,P) are connected by an edge.
Input Format :
The first line of input contains two space separated integers, that denotes the value of N and M.
Each of the following M lines contain two integers, that denote the vertices which have an undirected edge between them. Let us denote the two vertices with the symbol u and v.
Output Format :
Print the count the number of 3-cycles in the given graph
Constraints :
0 <= N <= 100
0 <= M <= (N*(N-1))/2
0 <= u <= N – 1
0 <= v <= N – 1
Time Limit: 1 sec
Sample Input 1:
3 3
0 1
1 2
2 0
Sample Output 1:
1
Coding ninjas solutions
Java Language [Solutions]
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
public class Solution {
static BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
public static int solve(boolean[][] graph, int n) {
int count=0;
for(int i=0;i<graph.length;i++) {
for(int j=0;j<graph.length;j++) {
if(graph[i][j] ==true) {
for(int k=0;k<graph.length;k++) {
if(k!=i && graph[k][j]==true && graph[i][k] ==true ) {
count++;
}
}
}
}
}
return count/6; //here we are doing count/6, because we are not using visited array,
//so 1 cycle is counted 2 times for each of the three vertices, one in clockwise one in anti clockwise
}
public static boolean[][] takeInput() throws IOException {
String[] strNums;
strNums = br.readLine().split("\\s");
int n = Integer.parseInt(strNums[0]);
int m = Integer.parseInt(strNums[1]);
boolean[][] graphs = new boolean[n][n];
int firstvertex, secondvertex;
for (int i = 0; i < m; i++) {
String[] strNums1;
strNums1 = br.readLine().split("\\s");
firstvertex = Integer.parseInt(strNums1[0]);
secondvertex = Integer.parseInt(strNums1[1]);
graphs[firstvertex][secondvertex] = true;
graphs[secondvertex][firstvertex] = true;
}
return graphs;
}
public static void main(String[] args) throws NumberFormatException, IOException {
boolean[][] graphs = takeInput();
int ans = solve(graphs, graphs.length);
System.out.println(ans);
}
}
Conclusion
Coding Ninja primarily targets students and professionals looking to enhance their coding and programming skills, prepare for technical interviews, or pursue careers in software development and related fields. It has gained a reputation for its quality courses and experienced instructors, making it a popular choice among those looking to improve their coding abilities.
Related Articles:
- About coding ninja questions
- Rectangular Numbers Coding Ninjas questions with solution
- How to Install Flutter in windows 10
- How to Setup Space Between Elements In Flutter
- Flutter Card Widget with Example
- Integrating an API into a Flutter – Working with REST APIs
- Create Login Page UI Design and Animation For Flutter