Android Game Development tutorial
In this android game Development tutorial we are going to create a amazing 2D Flying fish game app with source code free and provide a YouTube video .The Flying Fish Game is Simple and very Sweet Game to play For kids and Everyone
About Flying fish game
1.Flying Fish is back with new ways to play game.
Its an amazing game which will help u to increase your concentration and
In this game you will able to see the 3 balls but in which the blue and green ball is helpful for getting score and black ball is negative.
The original mode is back with improved physics and other refinements.In this android game Development tutorial Jump through three rings in a row to get a shot at a bonus ring for extra points.
Again Triple Ring Mode – The title says it all, there are three times the amount of rings and three times the amount of points!
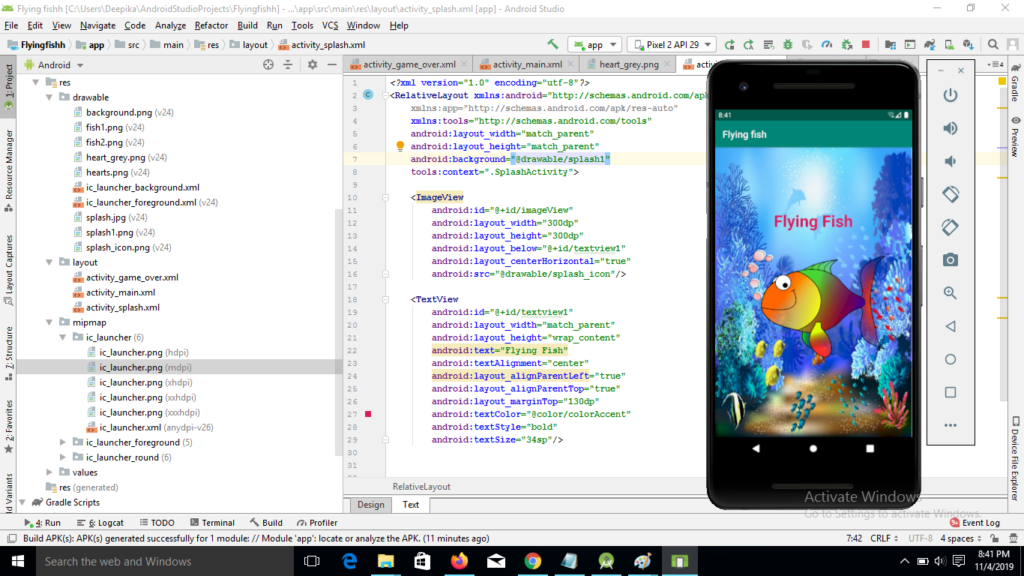
There are also new and returning skins, all completely redrawn to look better than ever. so try to unlock the new themes! and These change your background to better fit your preference , Because this android flying fish game is very interesting. so let’s play our game and how to make flying fish app.
MainActivity.java
package com.deep.flyingfishh; import androidx.appcompat.app.AppCompatActivity; import android.os.Bundle; import android.os.Handler; import java.util.Timer; import java.util.TimerTask; public class MainActivity extends AppCompatActivity { private FlyingFishView gameView; private Handler handler = new Handler(); private final static long Interval = 30; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); gameView = new FlyingFishView(this); setContentView(gameView); Timer timer = new Timer(); timer.schedule(new TimerTask() { @Override public void run() { handler.post(new Runnable() { @Override public void run() { gameView.invalidate(); } }); } }, 0, Interval); } }
Android game development This is Flying Fish View Source Code
FlyingFishView.java
package com.deep.flyingfishh; import android.content.Context; import android.content.Intent; import android.graphics.Bitmap; import android.graphics.BitmapFactory; import android.graphics.Canvas; import android.graphics.Color; import android.graphics.Paint; import android.graphics.Typeface; import android.view.MotionEvent; import android.view.View; import android.widget.Toast; public class FlyingFishView extends View { private Bitmap fish[] = new Bitmap[2]; private int fishX = 10; private int fishY; private int fishSpeed; private int canvasWidth, canvasHeight; private int yellowX, yellowY, yellowSpeed = 16; private Paint yellowPaint = new Paint(); private int greenX, greenY, greenSpeed = 20; private Paint greenPaint = new Paint(); private int redX, redY, redSpeed = 25; private Paint redPaint = new Paint(); private int score, lifeCounterOfFish; private boolean touch = false; private Bitmap backgroundImage; private Paint scorePaint = new Paint(); private Bitmap life[] = new Bitmap[2]; public FlyingFishView(Context context) { super(context); fish[0] = BitmapFactory.decodeResource(getResources(), R.drawable.fish1); fish[1] = BitmapFactory.decodeResource(getResources(), R.drawable.fish2); backgroundImage = BitmapFactory.decodeResource(getResources(), R.drawable.background); yellowPaint.setColor(Color.YELLOW); yellowPaint.setAntiAlias(false); greenPaint.setColor(Color.GREEN); greenPaint.setAntiAlias(false); redPaint.setColor(Color.RED); redPaint.setAntiAlias(false); scorePaint.setColor(Color.WHITE); scorePaint.setTextSize(70); scorePaint.setTypeface(Typeface.DEFAULT_BOLD); scorePaint.setAntiAlias(true); life[0] = BitmapFactory.decodeResource(getResources(), R.drawable.hearts); life[1] = BitmapFactory.decodeResource(getResources(), R.drawable.heart_grey); fishY = 550; score = 0; lifeCounterOfFish = 3; } @Override protected void onDraw(Canvas canvas) { super.onDraw(canvas); canvasWidth = canvas.getWidth(); canvasHeight = canvas.getHeight(); canvas.drawBitmap(backgroundImage, 0, 0, null); int minFishY = fish[0].getHeight(); int maxFishY = canvasHeight - fish[0].getHeight() * 3; fishY = fishY + fishSpeed; if (fishY < minFishY) { fishY = minFishY; } if (fishY > maxFishY) { fishY = maxFishY; } fishSpeed = fishSpeed + 2; if (touch) { canvas.drawBitmap(fish[1], fishX, fishY, null); touch = false; } else { canvas.drawBitmap(fish[0], fishX, fishY, null); } yellowX = yellowX - yellowSpeed; if (hitBallChecker(yellowX, yellowY)) { score = score + 10; yellowX = -100; } if (yellowX < 0) { yellowX = canvasWidth + 21; yellowY = (int) Math.floor(Math.random() * (maxFishY - minFishY)) + minFishY; } canvas.drawCircle(yellowX, yellowY, 25, yellowPaint); greenX = greenX - greenSpeed; if (hitBallChecker(greenX, greenY)) { score = score + 20; greenX = -100; } if (greenX < 0) { greenX = canvasWidth + 21; greenY = (int) Math.floor(Math.random() * (maxFishY - minFishY)) + minFishY; } canvas.drawCircle(greenX, greenY, 25, greenPaint); redX = redX - redSpeed; if (hitBallChecker(redX, redY)) { redX = -100; lifeCounterOfFish--; if (lifeCounterOfFish == 0) { Toast.makeText(getContext(), "Game Over", Toast.LENGTH_SHORT).show(); Intent gameOverIntent = new Intent(getContext(), GameOverActivity.class); gameOverIntent.addFlags(Intent.FLAG_ACTIVITY_NEW_TASK | Intent.FLAG_ACTIVITY_CLEAR_TASK); gameOverIntent.putExtra("score", score); getContext().startActivity(gameOverIntent); } } if (redX < 0) { redX = canvasWidth + 21; redY = (int) Math.floor(Math.random() * (maxFishY - minFishY)) + minFishY; } canvas.drawCircle(redX, redY, 30, redPaint); canvas.drawText("Score : " + score, 20, 60, scorePaint); for (int i = 0; i < 3; i++) { int x = (int) (580 + life[0].getWidth() * 1.5 * i); int y = 30; if (i < lifeCounterOfFish) { canvas.drawBitmap(life[0], x, y, null); } else { canvas.drawBitmap(life[1], x, y, null); } } } public boolean hitBallChecker(int x, int y) { if (fishX < x && x < (fishX + fish[0].getWidth()) && fishY < y && y < (fishY + fish[0].getHeight())) { return true; } return false; } @Override public boolean onTouchEvent(MotionEvent event) { if (event.getAction() == MotionEvent.ACTION_DOWN) { touch = true; fishSpeed = -22; } return true; } }
SplashActivity.java
package com.deep.flyingfishh; import androidx.appcompat.app.AppCompatActivity; import android.content.Intent; import android.os.Bundle; public class SplashActivity extends AppCompatActivity { @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_splash); Thread thread = new Thread() { @Override public void run() { try { sleep(5000); } catch (Exception e) { e.printStackTrace(); } finally { Intent mainIntent = new Intent(SplashActivity.this, MainActivity.class); startActivity(mainIntent); } } }; thread.start(); } @Override protected void onPause() { super.onPause(); finish(); } }

This is Source Code of Game Over Activity Code
GameOverActivity.java
package com.deep.flyingfishh; import androidx.appcompat.app.AppCompatActivity; import android.content.Intent; import android.os.Bundle; import android.view.View; import android.widget.Button; import android.widget.TextView; public class GameOverActivity extends AppCompatActivity { private Button StartGameAgain; private TextView DisplayScore; private String score; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_game_over); score = getIntent().getExtras().get("score").toString(); StartGameAgain = (Button) findViewById(R.id.play_again_btn); DisplayScore = (TextView) findViewById(R.id.displayScore); StartGameAgain.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View view) { Intent mainIntent = new Intent(GameOverActivity.this, MainActivity.class); startActivity(mainIntent); } }); DisplayScore.setText("Score= " + score); } }
————————————————————————————————————————–
this is android game development XML file source code
activity_main.xml
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context=".MainActivity"> </RelativeLayout>
activity_splash.xml
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:background="@drawable/splash1" tools:context=".SplashActivity"> <ImageView android:id="@+id/imageView" android:layout_width="300dp" android:layout_height="300dp" android:layout_below="@+id/textview1" android:layout_centerHorizontal="true" android:src="@drawable/splash_icon"/> <TextView android:id="@+id/textview1" android:layout_width="match_parent" android:layout_height="wrap_content" android:text="Flying Fish" android:textAlignment="center" android:layout_alignParentLeft="true" android:layout_alignParentTop="true" android:layout_marginTop="130dp" android:textColor="@color/colorAccent" android:textStyle="bold" android:textSize="34sp"/> </RelativeLayout>
activity_game_over.xml
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:background="@drawable/splash1" tools:context=".GameOverActivity"> <TextView android:id="@+id/gameOver" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_alignParentLeft="true" android:layout_alignParentTop="true" android:layout_marginTop="80dp" android:text="Game Over" android:textAlignment="center" android:textColor="@color/colorAccent" android:textSize="70dp" android:textStyle="bold" /> <Button android:id="@+id/play_again_btn" android:layout_width="200dp" android:layout_height="wrap_content" android:layout_below="@+id/gameOver" android:layout_centerHorizontal="true" android:background="@color/colorAccent" android:padding="10dp" android:text="Play Again" android:textAllCaps="false" android:textColor="@android:color/background_light" android:textSize="18sp" android:textStyle="bold" /> <TextView android:id="@+id/displayScore" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_marginTop="450dp" android:text="score" android:textAlignment="center" android:textAppearance="@style/TextAppearance.AppCompat.Small" android:textColor="#F44336" android:textSize="36sp" android:textStyle="bold" /> </RelativeLayout>
——————————————————————————————————————
Manifest File Source Code
AndroidManifest.xml
<?xml version="1.0" encoding="utf-8"?> <manifest xmlns:android="http://schemas.android.com/apk/res/android" package="com.deep.flyingfishh"> <application android:allowBackup="true" android:icon="@mipmap/ic_launcher" android:label="@string/app_name" android:roundIcon="@mipmap/ic_launcher_round" android:supportsRtl="true" android:theme="@style/AppTheme"> <activity android:name=".GameOverActivity"></activity> <activity android:name=".MainActivity"></activity> <activity android:name=".SplashActivity"> <intent-filter> <action android:name="android.intent.action.MAIN" /> <category android:name="android.intent.category.LAUNCHER" /> </intent-filter> </activity> </application> </manifest>
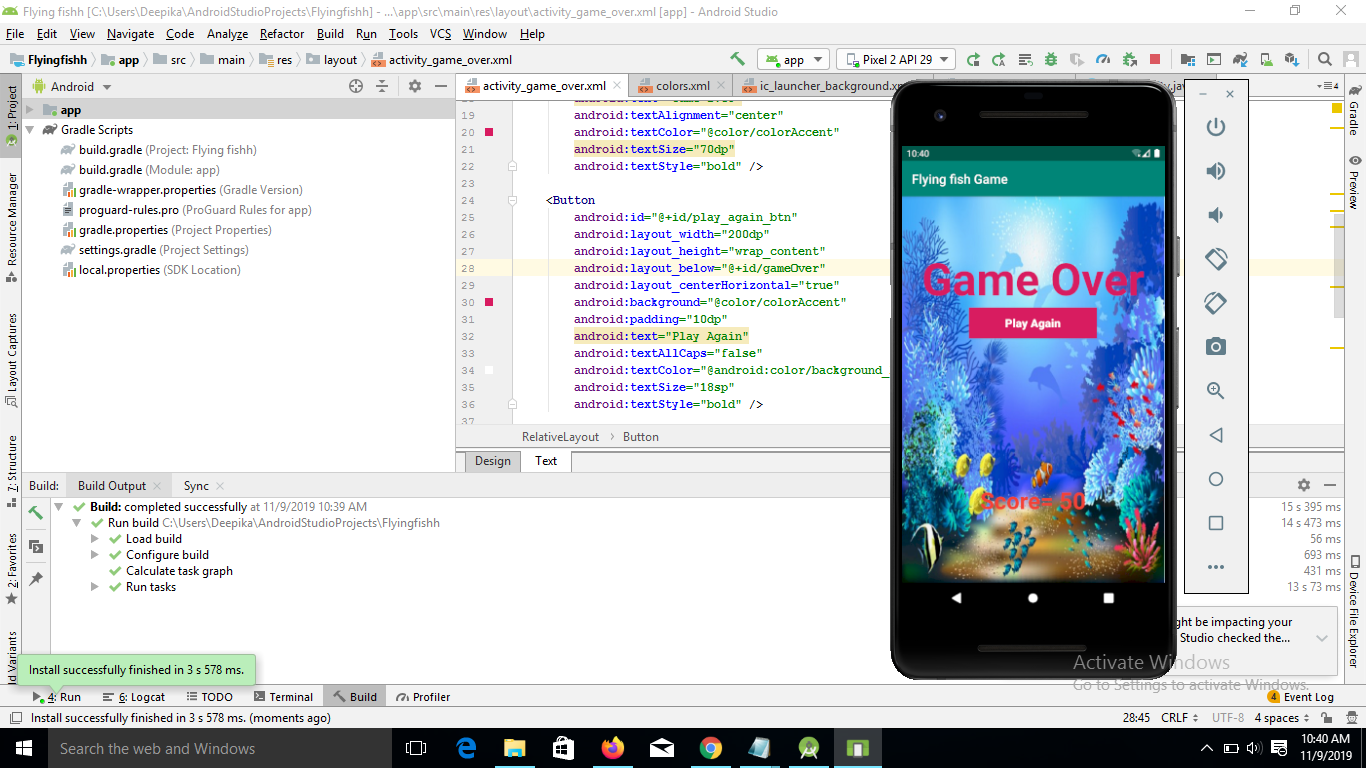
res > values > colors.xml
<?xml version="1.0" encoding="utf-8"?> <resources> <color name="colorPrimary">#008577</color> <color name="colorPrimaryDark">#00574B</color> <color name="colorAccent">#D81B60</color> </resources>
Visit my Google Play Store Apps
Visit For Browser 5 G App On google Play Store>>>>>>>>>>
This My Creative App Click on DeepCrazyWorld App>>>>>>>>>>>
To Solve Your Math Calculation Math Solve App >>>>>
Spinner Bottle Game App>>>>>>>>>>>>>>>>>>>>>
This News Nation App News7on>>>>>>>>>>>>>>
Shopping App ZampKart >>>>>>>>>>>>>>>>>>>
Math Equation Solving App>>>>>>>>>>>>>>>>>>>
Event Basis Picture LovingCaring143 App>>>>>>>>>
Here This Blogger Site App to Explore Your Knowledge Download all this Apps By Google Play Store My Blogger Site App Download And InstallParagraph
Click on Link CrazyCoder>>>>>>>>>>
Flying Fish YouTube Video
Get The Flying Fish Full Source Code
Click below to get the full source code android application.
Flying Fish game Images Download Click Here.
Conclusion
We have successfully created a android game development- Flying Fish game Android application using Android Studio.