Top 10 Flutter Interview Questions in 2023 – Creating a mobile application is often a difficult and demanding undertaking. For the development of mobile applications, there are several frameworks available.
Introduction
The native frameworks offered by iOS and Android are based on the Objective-C/Swift programming languages, respectively. But, we must write in two distinct languages and use two different frameworks in order to create an application that supports both OSs. Mobile frameworks supporting both OS exist to aid in overcoming this complication. These frameworks range from straightforward HTML-based hybrid mobile application frameworks (which employ JavaScript for application logic and HTML for user interface) to intricate language-specific frameworks (which do the heavy lifting of converting code to native code).

Q1: What is Flutter?
Answer: Flutter is an open-source UI toolkit from Google for crafting beautiful, natively compiled applications for desktop, web, and mobile from a single codebase. Flutter apps are built using the Dart programming language to create applications. Flutter’s first alpha version was released in 2017. Flutter is optimized for 2D mobile applications that execute on both iOS and Android platforms.
Following are the main elements of Flutter:
- Flutter engine
- Dart platform
- Design-specific widgets
- Foundation Library
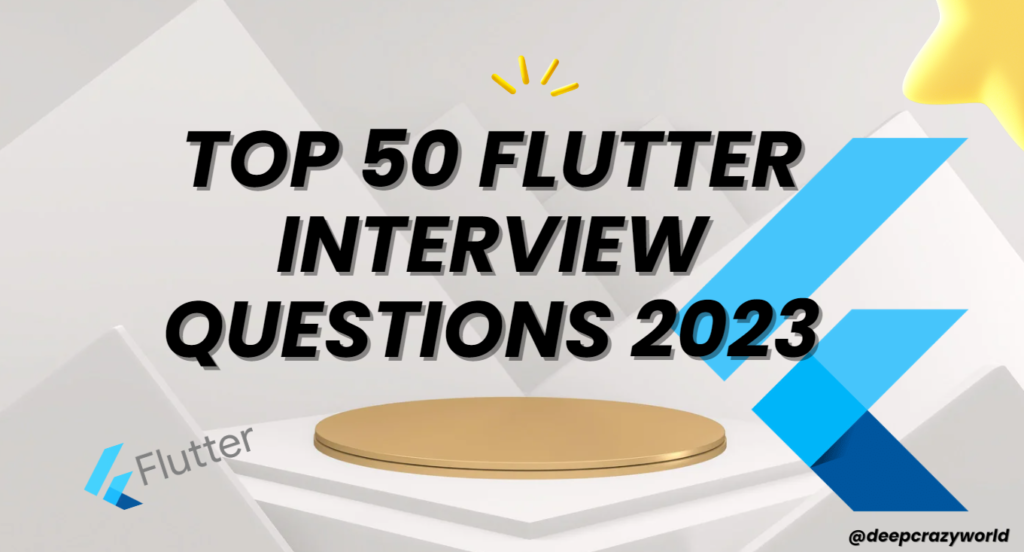
Q 2: When to use main Axis Alignment and cross Axis Alignment?
Answer
For Row:
mainAxisAlignment = Horizontal Axis
crossAxisAlignment = Vertical Axis
For Column:
mainAxisAlignment = Vertical Axis
crossAxisAlignment = Horizontal Axis

Q3: What is the difference between Expanded and Flexible widgets?
Answer: Expanded is just a shorthand for Flexible
Using expanded this way:
Expanded(
child: Foo(),
);
is strictly equivalent to:
Flexible(
fit: FlexFit.tight,
child: Foo(),
);
You may want to use Flexible over Expanded when you want a different fit, useful in some responsive layouts.
The difference between FlexFit.tight and FlexFit.loose is that loose will allow its child to have a maximum size while tight forces that child to fill all the available space.
Q4: What is the pubspec.yaml file and what does it do?
- Answer: The pubspec.yaml file allows you to define the packages your app relies on, declare your assets like images, audio, video, etc.
- It allows you to set constraints for your app.
- For Android developers, this is roughly similar to a build.gradle file.
Q5: Does Flutter work like a browser? How is it different from a WebView based application?
Answer: Simply said, to answer your question: A WebView or comparable app requires numerous levels of processing before the code you write can be executed. Flutter essentially outperforms that by compiling to native ARM code that runs on both systems. “Hybrid” apps operate slowly, have a clunky interface, and don’t match the platform they’re designed for. The performance of Flutter apps is noticeably faster than that of hybrid apps. Also, using plugins rather than WebViews, which are limited in how much of their platform they can utilise, makes it much simpler to access native components and sensors.
Q6: When should you use WidgetsBindingObserver?
Answer: WidgetsBindingObserver should be used when we want to listen to the AppLifecycleState and call stop/start on our services.
Q7: What is the difference between main() and runApp() functions in Flutter?
Answer:
main () function came from Java-like languages so it’s where all program started, without it, you can’t write any program on Flutter even without UI.
runApp() function should return Widget that would be attached to the screen as a root of the Widget Tree that will be rendered.
Q8: What is an App state?
Answer: State that is not ephemeral, that you want to share across many parts of your app, and that you want to keep between user sessions, is what we call application state (sometimes also called shared state).
Examples of application state: –
- User preferences –
- Login info –
- Notifications in a social networking app –
- The shopping cart in an e-commerce app –
- Read/unread state of articles in a news app
Q9: What are the different build modes in Flutter?
Answer: The Flutter tooling supports three modes when compiling your app, and a headless mode for testing.
You choose a compilation mode depending on where you are in the development cycle.
The modes are: – Debug – Profile – Release
Q10: What is Dart and why does Flutter use it?
Answer: Dart is an object-oriented, garbage-collected programming language that you use to develop Flutter apps. It was also created by Google, but is open-source, and has community inside and outside Google. Dart was chosen as the language of Flutter for the following reason:
Dart is AOT (Ahead Of Time) compiled to fast, predictable, native code, which allows almost all of Flutter to be written in Dart. This not only makes Flutter fast, virtually everything (including all the widgets) can be customized.
Dart can also be JIT (Just In Time) compiled for exceptionally fast development cycles and game-changing workflow (including Flutter’s popular sub-second stateful hot reload).
Dart allows Flutter to avoid the need for a separate declarative layout language like JSX or XML, or separate visual interface builders, because Dart’s declarative, programmatic layout is easy to read and visualize. And with all the layout in one language and in one place, it is easy for Flutter to provide advanced tooling that makes layout a snap.
Q11: How many types of widgets are there in Flutter?
Answer: There are two types of widgets:
- StatelessWidget : A widget that does not require mutable state. we can not change states.
- StatefulWidget: A widget that has mutable state. we can change there states.
Conclusion
These Flutter Interview questions of 2023, will provide you with a perception of the type of questions that can be asked in the Flutter job interview. If you have any queries, let us know by commenting in the below section.
Related Articles:
- How to Install Flutter in windows 10
- How to Setup Space Between Elements In Flutter
- Flutter Card Widget with Example
- Integrating an API into a Flutter – Working with REST APIs
- Create a simple splash screen in Flutter
- Android Projects with Source Code
- Flutter Interview Questions
- School Database Management System Project
- Create A Simple Splash Screen UI design
- Create Login Page UI Design and Animation For Flutter